- A pure-Python implementation of the AES block cipher algorithm and the common modes of operation (CBC, CFB, CTR, ECB and OFB).
- The following steps are involved in generating RSA keys −. Create two large prime numbers namely p and q. The product of these numbers will be called n, where n= p.q. Generate a random number which is relatively prime with (p-1) and (q-1). Let the number be called as e. Calculate the modular inverse of e.
AES (Advanced Encryption Standard) is a symmetric block cipher standardizedby NIST . It has a fixed data block size of 16 bytes.Its keys can be 128, 192, or 256 bits long.
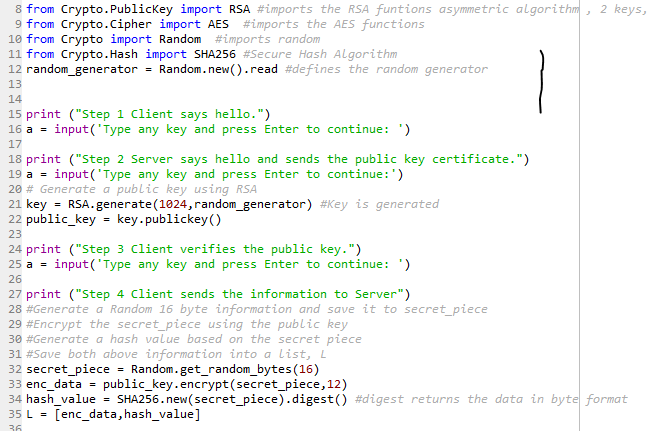
AES is very fast and secure, and it is the de facto standard for symmetricencryption.
Nwn2 Storm Of Zehir Cd Key Generator Aes Secret Key Generator Python Generate Non Expiring Api Key Jwt Ruby On Rails Vmware 7.1 License Key Generator Dpi The Key Technology For Next Generation Sd-wan Solutions Generate Wallet Address Without Private Key Dragon Ball Xenoverse Steam Key Generator Grid 2 Steam Key Generator. # Python AES implementation: import sys, hashlib, string, getpass: from copy import copy: from random import randint # The actual Rijndael specification includes variable block size, but # AES uses a fixed block size of 16 bytes (128 bits) # Additionally, AES allows for a variable key size, though this implementation # of AES uses only 256-bit.
As an example, encryption can be done as follows:
Random key generation; String encryption; Java version. Openjdk8; oraclejdk9; openjdk9; oraclejdk11; openjdk11; oraclejdk13; openjdk13; Example Code for Java String Encryption with key generation using AES-GCM.
The recipient can obtain the original message using the same keyand the incoming triple (nonce,ciphertext,tag)
:
Module’s constants for the modes of operation supported with AES:
var MODE_ECB: | Electronic Code Book (ECB) |
---|---|
var MODE_CBC: | Cipher-Block Chaining (CBC) |
var MODE_CFB: | Cipher FeedBack (CFB) |
var MODE_OFB: | Output FeedBack (OFB) |
var MODE_CTR: | CounTer Mode (CTR) |
var MODE_OPENPGP: | |
OpenPGP Mode | |
var MODE_CCM: | Counter with CBC-MAC (CCM) Mode |
var MODE_EAX: | EAX Mode |
var MODE_GCM: | Galois Counter Mode (GCM) |
var MODE_SIV: | Syntethic Initialization Vector (SIV) |
var MODE_OCB: | Offset Code Book (OCB) |
Crypto.Cipher.AES.
new
(key, mode, *args, **kwargs)¶Create a new AES cipher.
Parameters: |
|
---|---|
Keyword Arguments: | |
| |
Return: | an AES object, of the applicable mode. |
- Getting a Key
Using the cryptography module in Python, this post will look into methods of generating keys, storing keys and using the asymmetric encryption method RSA to encrypt and decrypt messages and files. We will be using cryptography.hazmat.primitives.asymmetric.rsa to generate keys.
Installing cryptography
Since Python does not come with anything that can encrypt files, we will need to use a third-party module.
PyCrypto is quite popular but since it does not offer built wheels, if you don't have Microsoft Visual C++ Build Tools installed, you will be told to install it. Instead of installing extra tools just to build this, I will be using the cryptography module. To install this, execute:
To make sure it installed correctly, open IDLE and execute:
If no errors appeared it has been installed correctly.
What is Asymmetric Encryption?
If you read my article on Encryption and Decryption in Python, you will see that I only used one key to encrypt and decrypt.
Asymmetric encryption uses two keys - a private key and a public key. Public keys are given out for anyone to use, you make them public information. Anyone can encrypt data with your public key and then only those with the private key can decrypt the message. This also works the other way around but it is a convention to keep your private key secret.
Aes Key Generation In Python 8
Getting a Key
To generate the two keys, we can call rsa.generate_private_key with some general parameters. The public key will be found in the object that holds the creation of the private key.
Storing Keys
To store the keys in a file, they first need to be serialized and then written to a file. To store the private key, we need to use the following.
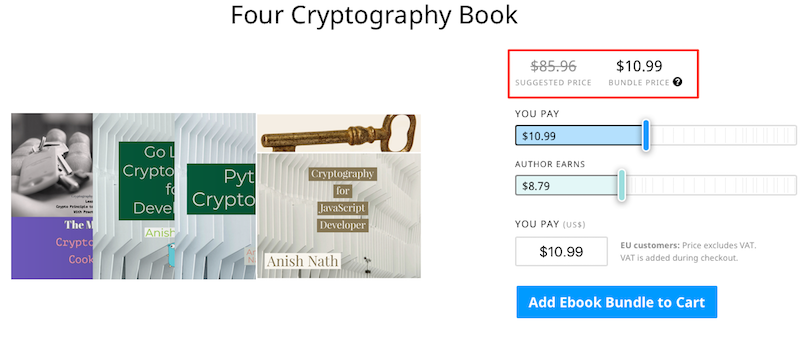
You can password protect the contents of this file using this top key serialization example.
To store the public key, we need to use a slightly modified version.
Remember that public and private keys are different so you will have to use these methods for each key.
Reading Keys
To get the keys out of the files, we need to read each file and then load them. To read the private key, use the following.
If you store the key with a password, set password to what you used.
The variable private_key will now have the private key. To read the public key, we need to use a slightly modified version.
The variable public_key will now have the public key.
Encrypting
Due to how asymmetric encryption algorithms like RSA work, encrypting with either one is fine, you just will need to use the other to decrypt. Applying a bit of logic to this can create some useful scenarios like signing and verification. For this example, I will assume that you keep both keys safe and don't release them since this example is only for local encryption (can be applied to wider though when keys are exchanged).
This means you can use either key but I will demonstrate using the public key to encrypt, this will mean anyone with the private key can decrypt the message.
Decrypting
Assuming that the public key was used to encrypt, we can use the private key to decrypt.
Demonstration
Aes Key Generation In Python 2
To show this in action, here is a properly constructed example.
Encrypting and Decrypting Files
To encrypt and decrypt files, you will need to use read and write binary when opening files. You can simply substitute the values I previously used for message
with the contents of a file. For example:
Using the variable message you can then encrypt it. To store, you can use the general Python method when encryption returns bytes.
Now to decrypt you can easily read the data from test.encrypted like the first bit of code in this section, decrypt it and then write it back out to test.txt using the second bit of code in this section.